http://mscoder.wordpress.com/2010/03/14/dynamically-set-the-autocompleteextender-contextkey/
Here in this article I will be explaining How to set and use
AutocompleteExtender’s ContextKey Dynamically to Load the data based on
some condition. I am assuming that you know how to use
AutoCompleteExtender Control of AJAX toolkit (
Please refer this link for basic info).
Basically this post is helpful for the below given problem.
Senerio – Let’s assume there is a textbox where we implement
autocompleteExtender to load Countries List, and there is one more
textbox for state but in state textbox we want to load the state list
based on country selected in first Textbox.
To overcome with the above problem we need to use ContextKey and need to change it dynamically.
Here is the example
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="MSCoderAutoCompleteKeyValuePair._Default" %>
<%@ Register Assembly="AjaxControlToolkit" Namespace="AjaxControlToolkit" TagPrefix="cc1" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<script type="text/javascript" language="javascript">
function SetCountryID(source, eventArgs) {
$find('StateAutoComplete').set_contextKey(eventArgs.get_value());
}
</script>
</head>
<body>
<form id="form1" runat="server">
<asp:ScriptManager ID="ScriptManager1" runat="server" />
<table>
<tr>
<td>
Country
</td>
<td>
<cc1:AutoCompleteExtender ID="CountryAutoComplete" runat="server" TargetControlID="txtCountry"
EnableCaching="false" CompletionSetCount="20" MinimumPrefixLength="1" ServicePath="wsAutoCompleteService.asmx"
FirstRowSelected="true" ServiceMethod="GetCountriesList" OnClientItemSelected="SetCountryID" />
<asp:TextBox ID="txtCountry" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td>
State
</td>
<td>
<cc1:AutoCompleteExtender ID="StateAutoComplete" runat="server" TargetControlID="txtState"
EnableCaching="false" MinimumPrefixLength="1" ServicePath="wsAutoCompleteService.asmx"
FirstRowSelected="true" ServiceMethod="GetStatesList" />
<asp:TextBox ID="txtState" runat="server"></asp:TextBox>
</td>
</tr>
</table>
</form>
</body>
</html>
Webservice to fetch the data from the Database
using System;
using System.Collections.Generic;
using System.Data.SqlClient;
using System.Web.Services;
namespace MSCoderAutoCompleteKeyValuePair
{
/// <summary>
/// Summary description for AutoCompleteService
/// </summary>
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
// To allow this Web Service to be called from script, using ASP.NET AJAX
[System.Web.Script.Services.ScriptService]
public class AutoCompleteService : System.Web.Services.WebService
{
/// <summary>
/// Method to get Countries List
/// </summary>
/// <param name="prefixText"></param>
/// <param name="count"></param>
/// <returns>String array which contains Countries name</returns>
[WebMethod(true)]
public string[] GetCountriesList(string prefixText, int count)
{
if (count == 0)
count = 10;
List<String> result = new List<string>();
using (SqlConnection connection = new SqlConnection(System.Configuration.ConfigurationManager.ConnectionStrings["ConnectionString"].ConnectionString))
{
connection.Open();
SqlCommand cmd = new SqlCommand(string.Format("Select Top {0} ID,Name from CountryTable Where Name Like '{1}%'",count, prefixText), connection);
SqlDataReader reader = cmd.ExecuteReader();
if (reader != null)
{
while (reader.Read())
{
result.Add(AutoCompleteItem(Convert.ToString(reader["Name"]), Convert.ToString(reader["ID"])));
}
}
}
return result.ToArray();
}
/// <summary>
/// Method to get States List
/// </summary>
/// <param name="prefixText"></param>
/// <param name="count"></param>
/// <param name="contextKey"></param>
/// <returns>String array which contains States name</returns>
[WebMethod(true)]
public string[] GetStatesList(string prefixText, int count,string contextKey)
{
List<String> result = new List<string>();
using (SqlConnection connection = new SqlConnection(System.Configuration.ConfigurationManager.ConnectionStrings["ConnectionString"].ConnectionString))
{
connection.Open();
SqlCommand cmd = new SqlCommand(string.Format("Select Top {0} ID,Name from StateTable Where Name Like '{1}%' And CountryID={2}", count, prefixText, contextKey), connection);
SqlDataReader reader = cmd.ExecuteReader();
if (reader != null)
{
while (reader.Read())
{
result.Add(AutoCompleteItem(Convert.ToString(reader["Name"]), Convert.ToString(reader["ID"])));
}
}
}
return result.ToArray();
}
/// <summary>
/// Method to get Formatted String value which can be used for KeyValue Pair for AutoCompleteExtender
/// </summary>
/// <param name="value"></param>
/// <param name="id"></param>
/// <returns>Returns string value which holds key and value in a specific format</returns>
private string AutoCompleteItem(string value, string id)
{
return string.Format("{{\"First\":\"{0}\",\"Second\":\"{1}\"}}", value, id);
}
}
}
Code Behind file which contains nothing
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace MSCoderAutoCompleteKeyValuePair
{
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
}
}
When we select India in Country textbox then in state textbox all the
India’s states will get listed and when we select USA as a country then
USA’s states will be listed.
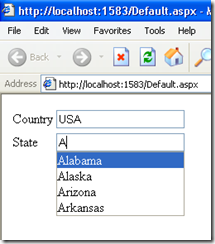
SQL Script for the Database
Create Database [Database]
Go
USE [Database]
Go
CREATE TABLE CountryTable(
[ID] int NOT NULL Primary key,
[Name] varchar(50) NOT NULL
)
Go
CREATE TABLE StateTable(
[ID] int NOT NULL Primary key,
[CountryID] int NOT NULL,
[Name] varchar(50) NOT NULL
)
Go
Insert Into CountryTable Values(1,'India')
Insert Into CountryTable Values(2,'USA')
Insert Into StateTable Values(1,1, 'Andhra Pradesh')
Insert Into StateTable Values(2,1, 'Arunachal Pradesh')
Insert Into StateTable Values(3,1, 'Assam')
Insert Into StateTable Values(4,1, 'Bihar')
Insert Into StateTable Values(5,1, 'Chhattisgarh')
Insert Into StateTable Values(6,1, 'Goa')
Insert Into StateTable Values(7,1, 'Gujarat')
Insert Into StateTable Values(8,1, 'Haryana')
Insert Into StateTable Values(9,1, 'Himachal Pradesh')
Insert Into StateTable Values(10,1, 'Jammu and Kashmir')
Insert Into StateTable Values(11,1, 'Jharkhand')
Insert Into StateTable Values(12,1, 'Karnataka')
Insert Into StateTable Values(13,1, 'Kerala')
Insert Into StateTable Values(14,1, 'Madhya Pradesh')
Insert Into StateTable Values(15,1, 'Maharashtra')
Insert Into StateTable Values(16,1, 'Manipur')
Insert Into StateTable Values(17,1, 'Meghalaya')
Insert Into StateTable Values(18,1, 'Mizoram')
Insert Into StateTable Values(19,1, 'Nagaland')
Insert Into StateTable Values(20,1, 'Orissa')
Insert Into StateTable Values(21,1, 'Punjab')
Insert Into StateTable Values(22,1, 'Rajasthan')
Insert Into StateTable Values(23,1, 'Sikkim')
Insert Into StateTable Values(24,1, 'Tamil Nadu')
Insert Into StateTable Values(25,1, 'Tripura')
Insert Into StateTable Values(26,1, 'Uttar Pradesh')
Insert Into StateTable Values(27,1, 'Uttarakhand')
Insert Into StateTable Values(28,1, 'West Bengal')
Insert Into StateTable Values(29,2, 'Alabama')
Insert Into StateTable Values(30,2, 'Alaska')
Insert Into StateTable Values(31,2, 'Arizona')
Insert Into StateTable Values(32,2, 'Arkansas')
Insert Into StateTable Values(33,2, 'California')
Insert Into StateTable Values(34,2, 'Colorado')
Insert Into StateTable Values(35,2, 'Connecticut')
Insert Into StateTable Values(36,2, 'Delaware')
Insert Into StateTable Values(37,2, 'Florida')
Insert Into StateTable Values(38,2, 'Georgia')
Insert Into StateTable Values(39,2, 'Hawaii')
Insert Into StateTable Values(40,2, 'Idaho')
Insert Into StateTable Values(41,2, 'Illinois')
Insert Into StateTable Values(42,2, 'Indiana')
Insert Into StateTable Values(43,2, 'Iowa')
Insert Into StateTable Values(44,2, 'Kansas')
Insert Into StateTable Values(45,2, 'Kentucky')
Insert Into StateTable Values(46,2, 'Louisiana')
Insert Into StateTable Values(47,2, 'Maine')
Insert Into StateTable Values(48,2, 'Maryland')
Insert Into StateTable Values(49,2, 'Massachusetts')
Insert Into StateTable Values(50,2, 'Michigan')
Insert Into StateTable Values(51,2, 'Minnesota')
Insert Into StateTable Values(52,2, 'Mississippi')
Insert Into StateTable Values(53,2, 'Missouri')
Insert Into StateTable Values(54,2, 'Montana')
Insert Into StateTable Values(55,2, 'Nebraska')
Insert Into StateTable Values(56,2, 'Nevada')
Insert Into StateTable Values(57,2, 'New Hampshire')
Insert Into StateTable Values(58,2, 'New Jersey')
Insert Into StateTable Values(59,2, 'New Mexico')
Insert Into StateTable Values(60,2, 'New York')
Insert Into StateTable Values(61,2, 'North Carolina')
Insert Into StateTable Values(62,2, 'North Dakota')
Insert Into StateTable Values(63,2, 'Ohio')
Insert Into StateTable Values(64,2, 'Oklahoma')
Insert Into StateTable Values(65,2, 'Oregon')
Insert Into StateTable Values(66,2, 'Pennsylvania')
Insert Into StateTable Values(67,2, 'Rhode Island')
Insert Into StateTable Values(68,2, 'South Carolina')
Insert Into StateTable Values(69,2, 'South Dakota')
Insert Into StateTable Values(70,2, 'Tennessee')
Insert Into StateTable Values(71,2, 'Texas')
Insert Into StateTable Values(72,2, 'Utah')
Insert Into StateTable Values(73,2, 'Vermont')
Insert Into StateTable Values(74,2, 'Virginia')
Insert Into StateTable Values(75,2, 'Washington')
Insert Into StateTable Values(76,2, 'West Virginia')
Insert Into StateTable Values(77,2, 'Wisconsin')
Insert Into StateTable Values(78,2, 'Wyo')